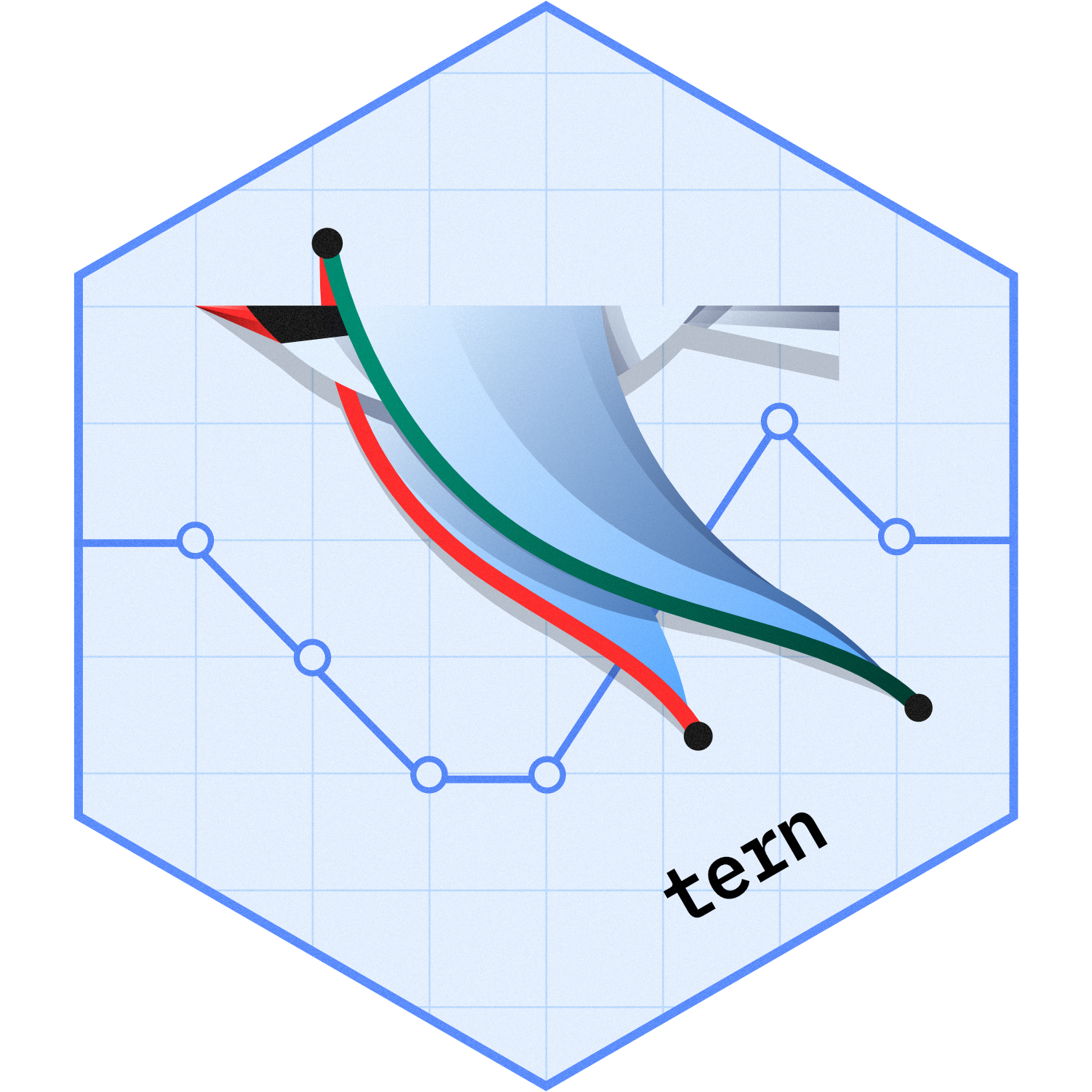
Count the number of patients with a particular event
Source:R/count_patients_with_event.R
count_patients_with_event.Rd
The analyze function count_patients_with_event()
creates a layout element to calculate patient counts for a
user-specified set of events.
This function analyzes primary analysis variable vars
which indicates unique subject identifiers. Events
are defined by the user as a named vector via the filters
argument, where each name corresponds to a
variable and each value is the value(s) that that variable takes for the event.
If there are multiple records with the same event recorded for a patient, only one occurrence is counted.
Usage
count_patients_with_event(
lyt,
vars,
filters,
riskdiff = FALSE,
na_str = default_na_str(),
nested = TRUE,
...,
table_names = vars,
.stats = "count_fraction",
.formats = list(count_fraction = format_count_fraction_fixed_dp),
.labels = NULL,
.indent_mods = NULL
)
s_count_patients_with_event(
df,
.var,
filters,
.N_col = ncol(df),
.N_row = nrow(df),
denom = c("n", "N_col", "N_row")
)
a_count_patients_with_event(
df,
labelstr = "",
filters,
.N_col,
.N_row,
denom = c("n", "N_col", "N_row"),
.df_row,
.var = NULL,
.stats = NULL,
.formats = NULL,
.labels = NULL,
.indent_mods = NULL,
na_str = default_na_str()
)
Arguments
- lyt
(
PreDataTableLayouts
)
layout that analyses will be added to.- vars
(
character
)
variable names for the primary analysis variable to be iterated over.- filters
(
character
)
a character vector specifying the column names and flag variables to be used for counting the number of unique identifiers satisfying such conditions. Multiple column names and flags are accepted in this formatc("column_name1" = "flag1", "column_name2" = "flag2")
. Note that only equality is being accepted as condition.- riskdiff
(
flag
)
whether a risk difference column is present. When set toTRUE
,add_riskdiff()
must be used assplit_fun
in the prior column split of the table layout, specifying which columns should be compared. Seestat_propdiff_ci()
for details on risk difference calculation.- na_str
(
string
)
string used to replace allNA
or empty values in the output.- nested
(
flag
)
whether this layout instruction should be applied within the existing layout structure _if possible (TRUE
, the default) or as a new top-level element (FALSE
). Ignored if it would nest a split. underneath analyses, which is not allowed.- ...
additional arguments for the lower level functions.
- table_names
(
character
)
this can be customized in the case that the samevars
are analyzed multiple times, to avoid warnings fromrtables
.- .stats
-
(
character
)
statistics to select for the table.Options are:
'n', 'count', 'count_fraction', 'count_fraction_fixed_dp', 'n_blq'
- .formats
(named
character
orlist
)
formats for the statistics. See Details inanalyze_vars
for more information on the"auto"
setting.- .labels
(named
character
)
labels for the statistics (without indent).- .indent_mods
(named
integer
)
indent modifiers for the labels. Defaults to 0, which corresponds to the unmodified default behavior. Can be negative.- df
(
data.frame
)
data set containing all analysis variables.- .var
(
string
)
name of the column that contains the unique identifier.- .N_col
(
integer(1)
)
column-wise N (column count) for the full column being analyzed that is typically passed byrtables
.- .N_row
(
integer(1)
)
row-wise N (row group count) for the group of observations being analyzed (i.e. with no column-based subsetting) that is typically passed byrtables
.- denom
-
(
string
)
choice of denominator for proportion. Options are:n
: number of values in this row and column intersection.N_row
: total number of values in this row across columns.N_col
: total number of values in this column across rows.
- labelstr
(
string
)
label of the level of the parent split currently being summarized (must be present as second argument in Content Row Functions). Seertables::summarize_row_groups()
for more information.- .df_row
(
data.frame
)
data frame across all of the columns for the given row split.
Value
count_patients_with_event()
returns a layout object suitable for passing to further layouting functions, or tortables::build_table()
. Adding this function to anrtable
layout will add formatted rows containing the statistics froms_count_patients_with_event()
to the table layout.
s_count_patients_with_event()
returns the count and fraction of unique identifiers with the defined event.
a_count_patients_with_event()
returns the corresponding list with formattedrtables::CellValue()
.
Functions
count_patients_with_event()
: Layout-creating function which can take statistics function arguments and additional format arguments. This function is a wrapper forrtables::analyze()
.s_count_patients_with_event()
: Statistics function which counts the number of patients for which the defined event has occurred.a_count_patients_with_event()
: Formatted analysis function which is used asafun
incount_patients_with_event()
.
Examples
lyt <- basic_table() %>%
split_cols_by("ARM") %>%
add_colcounts() %>%
count_values(
"STUDYID",
values = "AB12345",
.stats = "count",
.labels = c(count = "Total AEs")
) %>%
count_patients_with_event(
"SUBJID",
filters = c("TRTEMFL" = "Y"),
.labels = c(count_fraction = "Total number of patients with at least one adverse event"),
table_names = "tbl_all"
) %>%
count_patients_with_event(
"SUBJID",
filters = c("TRTEMFL" = "Y", "AEOUT" = "FATAL"),
.labels = c(count_fraction = "Total number of patients with fatal AEs"),
table_names = "tbl_fatal"
) %>%
count_patients_with_event(
"SUBJID",
filters = c("TRTEMFL" = "Y", "AEOUT" = "FATAL", "AEREL" = "Y"),
.labels = c(count_fraction = "Total number of patients with related fatal AEs"),
.indent_mods = c(count_fraction = 2L),
table_names = "tbl_rel_fatal"
)
build_table(lyt, tern_ex_adae, alt_counts_df = tern_ex_adsl)
#> A: Drug X B: Placebo C: Combination
#> (N=69) (N=73) (N=58)
#> ———————————————————————————————————————————————————————————————————————————————————————————————————
#> Total AEs 202 177 162
#> Total number of patients with at least one adverse event 59 (100%) 57 (100%) 48 (100%)
#> Total number of patients with fatal AEs 28 (47.5%) 31 (54.4%) 20 (41.7%)
#> Total number of patients with related fatal AEs 28 (47.5%) 31 (54.4%) 20 (41.7%)
s_count_patients_with_event(
tern_ex_adae,
.var = "SUBJID",
filters = c("TRTEMFL" = "Y"),
)
#> $n
#> n
#> 164
#>
#> $count
#> count
#> 164
#>
#> $count_fraction
#> count fraction
#> 164 1
#>
#> $count_fraction_fixed_dp
#> count fraction
#> 164 1
#>
#> $fraction
#> num denom
#> 164 164
#>
#> $n_blq
#> n_blq
#> 0
#>
s_count_patients_with_event(
tern_ex_adae,
.var = "SUBJID",
filters = c("TRTEMFL" = "Y", "AEOUT" = "FATAL")
)
#> $n
#> n
#> 164
#>
#> $count
#> count
#> 79
#>
#> $count_fraction
#> count fraction
#> 79.0000000 0.4817073
#>
#> $count_fraction_fixed_dp
#> count fraction
#> 79.0000000 0.4817073
#>
#> $fraction
#> num denom
#> 79 164
#>
#> $n_blq
#> n_blq
#> 0
#>
s_count_patients_with_event(
tern_ex_adae,
.var = "SUBJID",
filters = c("TRTEMFL" = "Y", "AEOUT" = "FATAL"),
denom = "N_col",
.N_col = 456
)
#> $n
#> n
#> 164
#>
#> $count
#> count
#> 79
#>
#> $count_fraction
#> count fraction
#> 79.0000000 0.1732456
#>
#> $count_fraction_fixed_dp
#> count fraction
#> 79.0000000 0.1732456
#>
#> $fraction
#> num denom
#> 79 456
#>
#> $n_blq
#> n_blq
#> 0
#>
a_count_patients_with_event(
tern_ex_adae,
.var = "SUBJID",
filters = c("TRTEMFL" = "Y"),
.N_col = 100,
.N_row = 100
)
#> RowsVerticalSection (in_rows) object print method:
#> ----------------------------
#> row_name formatted_cell indent_mod row_label
#> 1 n 164 0 n
#> 2 count 164 0 count
#> 3 count_fraction 164 (100%) 0 count_fraction
#> 4 count_fraction_fixed_dp 164 (100%) 0 count_fraction_fixed_dp
#> 5 n_blq 0 0 n_blq