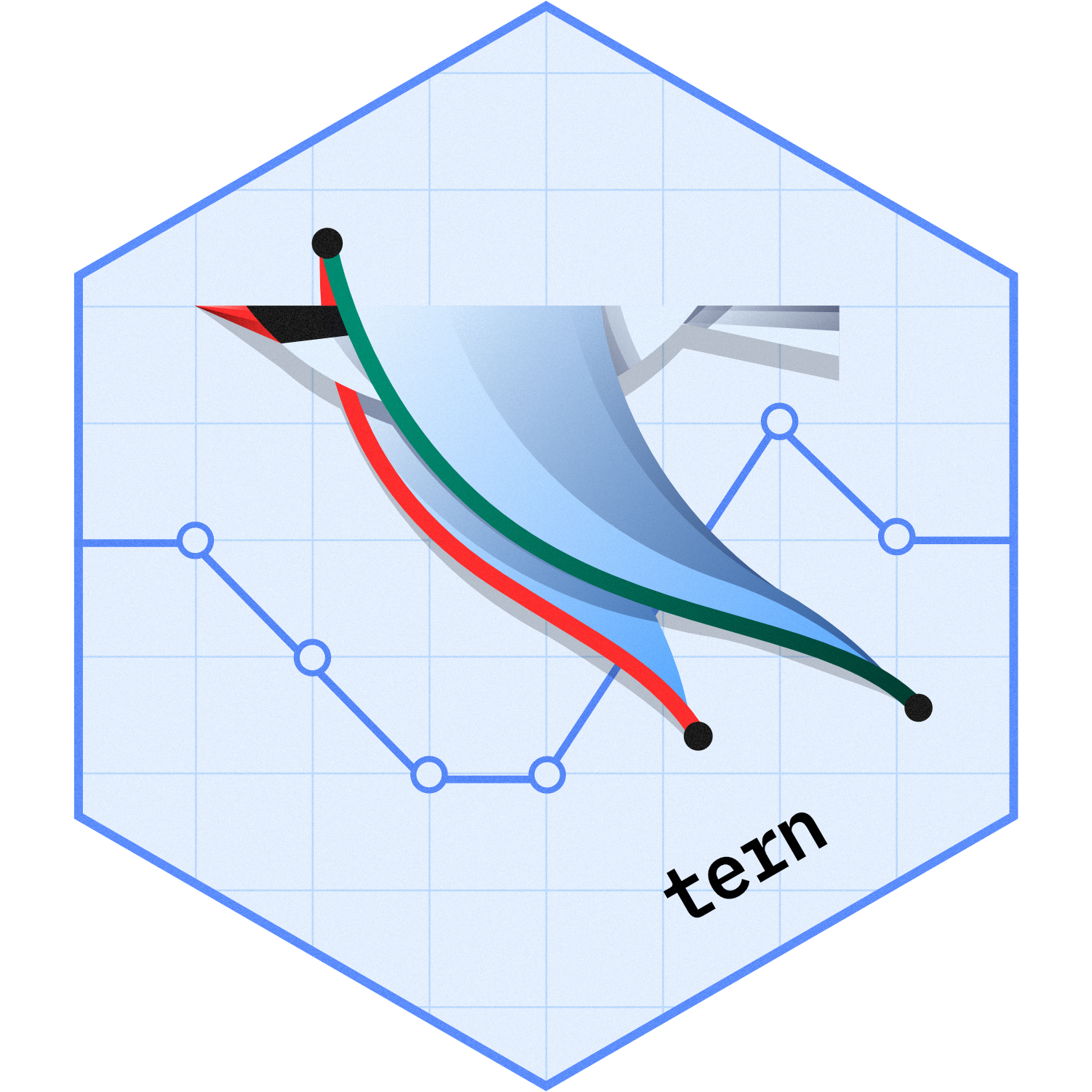
Helper functions for Cox proportional hazards regression
Source:R/h_cox_regression.R
h_cox_regression.Rd
Helper functions used in fit_coxreg_univar()
and fit_coxreg_multivar()
.
Usage
h_coxreg_univar_formulas(variables, interaction = FALSE)
h_coxreg_multivar_formula(variables)
h_coxreg_univar_extract(effect, covar, data, mod, control = control_coxreg())
h_coxreg_multivar_extract(var, data, mod, control = control_coxreg())
Arguments
- variables
(named
list
ofstring
)
list of additional analysis variables.- interaction
(
flag
)
ifTRUE
, the model includes the interaction between the studied treatment and candidate covariate. Note that for univariate models without treatment arm, and multivariate models, no interaction can be used so that this needs to beFALSE
.- effect
(
string
)
the treatment variable.- covar
(
string
)
the name of the covariate in the model.- data
(
data.frame
)
the dataset containing the variables to summarize.- mod
(
coxph
)
Cox regression model fitted bysurvival::coxph()
.- control
(
list
)
a list of controls as returned bycontrol_coxreg()
.- var
(
string
)
single variable name that is passed byrtables
when requested by a statistics function.
Value
h_coxreg_univar_formulas()
returns acharacter
vector coercible into formulas (e.gstats::as.formula()
).
h_coxreg_multivar_formula()
returns astring
coercible into a formula (e.gstats::as.formula()
).
h_coxreg_univar_extract()
returns adata.frame
with variableseffect
,term
,term_label
,level
,n
,hr
,lcl
,ucl
, andpval
.
h_coxreg_multivar_extract()
returns adata.frame
with variablespval
,hr
,lcl
,ucl
,level
,n
,term
, andterm_label
.
Functions
h_coxreg_univar_formulas()
: Helper for Cox regression formula. Creates a list of formulas. It is used internally byfit_coxreg_univar()
for the comparison of univariate Cox regression models.h_coxreg_multivar_formula()
: Helper for multivariate Cox regression formula. Creates a formulas string. It is used internally byfit_coxreg_multivar()
for the comparison of multivariate Cox regression models. Interactions will not be included in multivariate Cox regression model.h_coxreg_univar_extract()
: Utility function to help tabulate the result of a univariate Cox regression model.h_coxreg_multivar_extract()
: Tabulation of multivariate Cox regressions. Utility function to help tabulate the result of a multivariate Cox regression model for a treatment/covariate variable.
Examples
# `h_coxreg_univar_formulas`
## Simple formulas.
h_coxreg_univar_formulas(
variables = list(
time = "time", event = "status", arm = "armcd", covariates = c("X", "y")
)
)
#> ref
#> "survival::Surv(time, status) ~ armcd"
#> X
#> "survival::Surv(time, status) ~ armcd + X"
#> y
#> "survival::Surv(time, status) ~ armcd + y"
## Addition of an optional strata.
h_coxreg_univar_formulas(
variables = list(
time = "time", event = "status", arm = "armcd", covariates = c("X", "y"),
strata = "SITE"
)
)
#> ref
#> "survival::Surv(time, status) ~ armcd + strata(SITE)"
#> X
#> "survival::Surv(time, status) ~ armcd + X + strata(SITE)"
#> y
#> "survival::Surv(time, status) ~ armcd + y + strata(SITE)"
## Inclusion of the interaction term.
h_coxreg_univar_formulas(
variables = list(
time = "time", event = "status", arm = "armcd", covariates = c("X", "y"),
strata = "SITE"
),
interaction = TRUE
)
#> ref
#> "survival::Surv(time, status) ~ armcd + strata(SITE)"
#> X
#> "survival::Surv(time, status) ~ armcd * X + strata(SITE)"
#> y
#> "survival::Surv(time, status) ~ armcd * y + strata(SITE)"
## Only covariates fitted in separate models.
h_coxreg_univar_formulas(
variables = list(
time = "time", event = "status", covariates = c("X", "y")
)
)
#> X y
#> "survival::Surv(time, status) ~ 1 + X" "survival::Surv(time, status) ~ 1 + y"
# `h_coxreg_multivar_formula`
h_coxreg_multivar_formula(
variables = list(
time = "AVAL", event = "event", arm = "ARMCD", covariates = c("RACE", "AGE")
)
)
#> [1] "survival::Surv(AVAL, event) ~ ARMCD + RACE + AGE"
# Addition of an optional strata.
h_coxreg_multivar_formula(
variables = list(
time = "AVAL", event = "event", arm = "ARMCD", covariates = c("RACE", "AGE"),
strata = "SITE"
)
)
#> [1] "survival::Surv(AVAL, event) ~ ARMCD + RACE + AGE + strata(SITE)"
# Example without treatment arm.
h_coxreg_multivar_formula(
variables = list(
time = "AVAL", event = "event", covariates = c("RACE", "AGE"),
strata = "SITE"
)
)
#> [1] "survival::Surv(AVAL, event) ~ 1 + RACE + AGE + strata(SITE)"
library(survival)
dta_simple <- data.frame(
time = c(5, 5, 10, 10, 5, 5, 10, 10),
status = c(0, 0, 1, 0, 0, 1, 1, 1),
armcd = factor(LETTERS[c(1, 1, 1, 1, 2, 2, 2, 2)], levels = c("A", "B")),
var1 = c(45, 55, 65, 75, 55, 65, 85, 75),
var2 = c("F", "M", "F", "M", "F", "M", "F", "U")
)
mod <- coxph(Surv(time, status) ~ armcd + var1, data = dta_simple)
result <- h_coxreg_univar_extract(
effect = "armcd", covar = "armcd", mod = mod, data = dta_simple
)
result
#> effect term term_label level n hr lcl ucl
#> 1 Treatment: armcd B vs control (A) B 8 6.551448 0.4606904 93.16769
#> pval
#> 1 0.165209
mod <- coxph(Surv(time, status) ~ armcd + var1, data = dta_simple)
result <- h_coxreg_multivar_extract(
var = "var1", mod = mod, data = dta_simple
)
result
#> pval hr lcl ucl level n term term_label
#> 2 0.4456195 0.9423284 0.808931 1.097724 var1 8 var1 var1